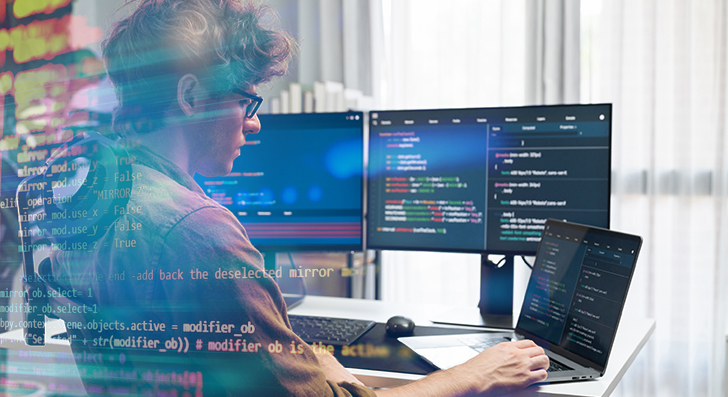
Scalability indicates your software can cope with expansion—a lot more customers, extra info, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Below’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be portion of your prepare from the beginning. A lot of applications fall short when they increase quickly for the reason that the initial structure can’t manage the additional load. As a developer, you must think early about how your procedure will behave stressed.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular layout or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale on its own with no affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of a million customers or simply 100? Choose the suitable type—relational or NoSQL—depending on how your knowledge will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath latest ailments. Give thought to what would happen In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-pushed devices. These enable your application take care of far more requests with no receiving overloaded.
Once you Construct with scalability in mind, you're not just making ready for achievement—you happen to be lowering potential headaches. A well-prepared technique is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is usually a critical Portion of developing scalable purposes. Not all databases are designed the identical, and using the Erroneous one can slow you down or maybe result in failures as your application grows.
Start out by knowing your data. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is a good in good shape. These are typically powerful with interactions, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle additional visitors and facts.
Should your details is more adaptable—like user action logs, product catalogs, or paperwork—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your browse and create styles. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could tackle substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also sensible to Assume in advance. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on database functionality while you increase.
Briefly, the appropriate databases relies on your application’s composition, pace demands, And just how you assume it to increase. Get time to choose wisely—it’ll save plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down functionality and overload your program. That’s why it’s vital that you Develop economical logic from the beginning.
Commence by creating clean, very simple code. Keep away from repeating logic and remove anything pointless. Don’t pick the most intricate Answer if a straightforward just one operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to search out bottlenecks—areas where your code can take also extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish items down much more than the code by itself. Be certain Every single question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout large tables.
In case you see the identical facts being requested time and again, use caching. Retailer the final results quickly utilizing instruments like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and helps make your application much more effective.
Remember to take a look at with significant datasets. Code and queries that work good with one hundred data could crash every time they have to take care of 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to deal with a lot more consumers and a lot more targeted traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to a single server performing all the work, the load balancer routes buyers to unique servers determined by availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused promptly. When end users request exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 popular forms of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching cuts down database load, enhances speed, and will make your app additional efficient.
Use caching for things which don’t change usually. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful resources. Jointly, they help your application tackle much more people, continue to be quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to rent servers and providers as you may need them. You don’t should invest in hardware or guess potential capability. When targeted traffic boosts, you may insert extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular Software for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If 1 part of your respective app crashes, it restarts it quickly.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update Gustavo Woltmann news or scale components independently, which happens to be great for general performance and dependability.
To put it briefly, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get well rapidly when challenges come about. If you want your application to mature without having restrictions, begin working with these tools early. They preserve time, cut down threat, and assist you stay focused on making, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when items go wrong. Monitoring will help the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by tracking primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and solutions are undertaking. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for users to load pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, Should your response time goes over a limit or a company goes down, you'll want to get notified straight away. This can help you deal with troubles quickly, usually prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again just before it leads to serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not just about recognizing failures—it’s about comprehending your process and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Get started tiny, Assume big, and Construct smart.